الخزانة الذكية
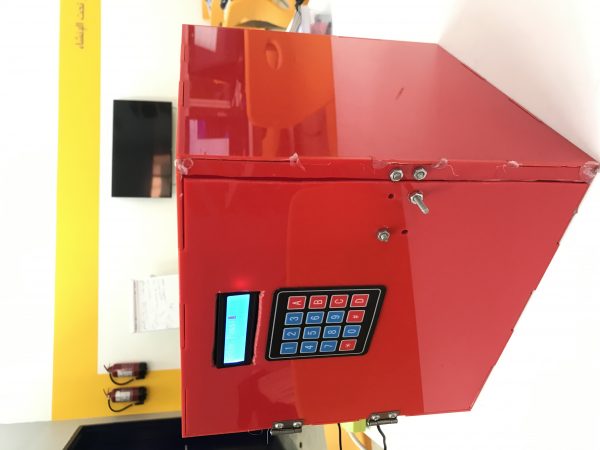
نبذة:
عبارة عن خزانة ذكية تفتح بستخدام كلمة مرورالادواة
الخزانة:-
مادة الكرليك
فصال حديد
قفل يدوي (نحاس)
القطع البرمجيه:-
Arduino Board – https://goo.gl/UyGYeF
Keypad 4×4 – https://goo.gl/jtGlx5
LCD 16×02 – https://goo.gl/8S27hc
Breadboard – https://goo.gl/yCa8hX
Potentiometer 10k – https://goo.gl/c90Uix
الكود البرمجي
//Start of Program
/*
|| Simple Password Entry Using Matrix Keypad
|| 4/5/2012 Updates Nathan Sobieck: Nathan@Sobisource.com
||
*/
//* is to validate password
//# is to reset password attempt
/////////////////////////////////////////////////////////////////
/* The circuit:
* LCD RS pin to digital pin 12
* LCD Enable pin to digital pin 11
* LCD D4 pin to digital pin 5
* LCD D5 pin to digital pin 4
* LCD D6 pin to digital pin 3
* LCD D7 pin to digital pin 2
* LCD R/W pin to ground
* LCD VSS pin to ground
* LCD VCC pin to 5V
* 10K resistor:
* ends to +5V and ground
* wiper to LCD VO pin (pin 3)
*/
#include <Password.h> //http://www.arduino.cc/playground/uploads/Code/Password.zip
#include <Keypad.h> //http://www.arduino.cc/playground/uploads/Code/Keypad.zip
#include <LiquidCrystal.h> //http://www.arduino.cc/en/Tutorial/LiquidCrystal
#include <Servo.h>
// initialize the library by associating any needed LCD interface pin
// with the arduino pin number it is connected to
const int rs = 12, en = 11, d4 = 5, d5 = 4, d6 = 3, d7 = 2;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
Password password = Password( "1234" );
int buz=13;
Servo myservo; // create servo object to control a servo
const byte ROWS = 4; // Four rows
const byte COLS = 4; // columns
// Define the Keymap
char keys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {A0,A1,A2,A3, };// Connect keypad ROW0, ROW1, ROW2 and ROW3 to these Arduino pins.
byte colPins[COLS] = { A4,A5,9,10, };// Connect keypad COL0, COL1 and COL2 to these Arduino pins.
int m1a=6;
int m1b=7;
// Create the Keypad
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
void setup()
{ myservo.write(7);
Serial.begin(9600);
lcd.begin(16, 2); // set up the LCD's number of columns and rows:
pinMode(buz,OUTPUT);
pinMode(m1a,OUTPUT);
pinMode(m1b,OUTPUT);
digitalWrite(buz, LOW);
lcd.setCursor(0, 0);
lcd.print("**Digital Door**");
lcd.setCursor(0, 1);
lcd.print("*Locking System*");
delay(2000);
lock();
keypad.addEventListener(keypadEvent); //add an event listener for this keypad
myservo.attach(8); // attaches the servo on pin 9 to the servo object
}
void loop(){
keypad.getKey();
}
//take care of some special events
void keypadEvent(KeypadEvent eKey){
switch (keypad.getState()){
case PRESSED:
lcd.print("*");
digitalWrite(buz, HIGH);
delay(100);
digitalWrite(buz, LOW);
switch (eKey){
case '*': checkPassword(); break;
case '#': lock(); break;
default: password.append(eKey);
}
}
}
void checkPassword(){
if (password.evaluate()) // Arduini will evaluate the keypress with the Actual Password
{
unlock();
}
else{
lcd.clear();
lcd.noBlink();
lcd.setCursor(0, 0);
lcd.print("*****Wrong*****");
lcd.setCursor(0, 1);
lcd.print("***************");
digitalWrite(buz, HIGH);
delay(100);
delay(1000);
digitalWrite(buz, LOW);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("***Try Again***");
delay(1000);
lcd.clear();
password.reset();
lcd.blink();
lcd.print("Enter Pass:");
}
}
void lock() // Function For Lock The Door
{
digitalWrite(m1a,HIGH);
digitalWrite(m1b,LOW);
delay(200);
digitalWrite(m1a,LOW);
digitalWrite(m1b,LOW);
lcd.clear();
lcd.setCursor(0, 0);
myservo.write(7);
lcd.print("**Door Locked**");
lcd.setCursor(0, 1);
lcd.print("***************");
delay(1000);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Etr Pas to Unlck");
lcd.setCursor(0, 1);
lcd.print("*****Again*****");
delay(2000);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Enter Pass:");
lcd.blink();
}
void unlock() // Function For Unlock The Door
{
lcd.clear();
lcd.noBlink();
lcd.setCursor(0, 0);
lcd.print("****Success****");
lcd.setCursor(0, 1);
lcd.print("***************");
myservo.write(58); // tell servo to go to position in variable 'pos'
digitalWrite(buz, HIGH);
digitalWrite(m1a,LOW);
digitalWrite(m1b,HIGH);
delay(200);
digitalWrite(m1a,LOW);
digitalWrite(m1b,LOW);
digitalWrite(buz, LOW);
delay(1000);
lcd.clear();
lcd.setCursor(0, 0);
lcd.noBlink();
lcd.print(" Press # key to");
lcd.setCursor(0, 1);
lcd.print("***Lock Again***");
password.reset();
}
// End of program
ممتاز و مبدع يا عبدالرحمن .. استمر بتطوير مهاراتك ،،
مشروع رائع جداً 👏🏻
دائما مبدع يا عبدالرحمن .. منها للأعلى
ابداع ماشاء الله تبارك الله